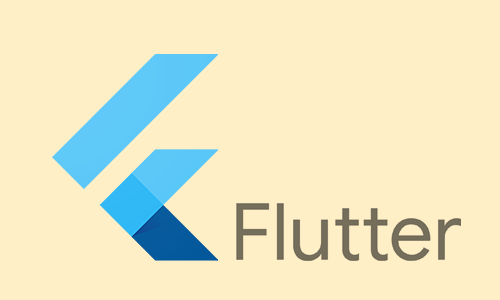
[Flutter] 위젯(Widget) 기초 - text, icon, button 등등
leteu
·2023. 3. 4. 21:17
써본 위젯 다 쓰진 못하겠고 많이 사용할만한 거만 계속 적어보려 한다.
#1 위젯 종류
https://flutter-ko.dev/docs/reference/widgets
Flutter 위젯 목록
flutter-ko.dev
위젯 종류가 굉장히 많다.
버튼만 해도 아이콘이 있고 없고 배경이 채워진 버튼, 아웃라인만 있는 버튼 등등 종류가 많다.
위 링크의 공식 문서 보면서 잠깐 개발해보며 많이 사용한 위젯 위주로 설명해보려고 한다.
#2 기초
위젯은 크게 두가지로 나뉜다.
##Stateful Widget
- 동적
- State의 데이터가 변할때마다 다시 빌드하는 위젯
- 상수는 가질 수 있지만 프로퍼티로 변수를 가지지 않음
- stful 치고 엔터누르면 바로 나온다.
클래스 명을 입력하면 알아서 코드가 다 나온다.
##Stateless Widget
- 정적
- 상태변화가 없는 위젯
- Stateful과 비슷하게 stless 치고 엔터누르면 자동으로 뽑아준다.
#3 Text
글자 위젯이다. 텍스트 넣고 싶으면 이거로 넣어야 한다.
Text('텍스트')
예제 코드
class Mainlayout extends StatefulWidget {
const Mainlayout({Key? key}) : super(key: key);
@override
_MainlayoutState createState() => _MainlayoutState();
}
class _MainlayoutState extends State<Mainlayout> {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Text('텍스트'),
),
);
}
}
#4 Icon
아이콘 위젯이다.
Icon 위젯 안에 아이콘을 넣어서 표출할 수 있다.
Icons.<아이콘 이름>
Icon(Icons.flutter_dash)
예제 코드
class Mainlayout extends StatefulWidget {
const Mainlayout({Key? key}) : super(key: key);
@override
_MainlayoutState createState() => _MainlayoutState();
}
class _MainlayoutState extends State<Mainlayout> {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Icon(Icons.flutter_dash, size: 150,),
),
);
}
}
#5 Button
버튼 위젯인데 종류가 너무 많다.
그냥 한 번에 다 보여줄 테니 원하는 거 긁어가는 게 편하다.
예제 코드
class Mainlayout extends StatefulWidget {
const Mainlayout({Key? key}) : super(key: key);
@override
_MainlayoutState createState() => _MainlayoutState();
}
class _MainlayoutState extends State<Mainlayout> {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Column(
children: [
const SizedBox(height: 20),
TextButton(
child: const Text('버튼1'),
onPressed: () {},
),
TextButton.icon(
onPressed: () {},
label: const Text('버튼2'),
icon: const Icon(Icons.add),
),
ElevatedButton(onPressed: () {}, child: const Text('버튼3')),
OutlinedButton(onPressed: () {}, child: const Text('버튼4')),
FloatingActionButton(onPressed: () {}, child: const Text('버튼5')),
FloatingActionButton.extended(
onPressed: () {},
label: const Text('버튼6'),
icon: const Icon(Icons.add)
),
CupertinoButton(
onPressed: () {},
child: const Text('버튼7'),
),
CupertinoButton.filled(
onPressed: () {},
child: const Text('버튼8'),
),
],
),
),
);
}
}
'프로그래밍 > Flutter' 카테고리의 다른 글
[Flutter] 위젯(Widget) 알아보기 - Scaffold (0) | 2023.03.04 |
---|---|
[Flutter] Flutter 1 - 설치부터 빠르게 (0) | 2023.03.04 |
[Flutter] API 불러오고 사용하기 (0) | 2022.01.25 |